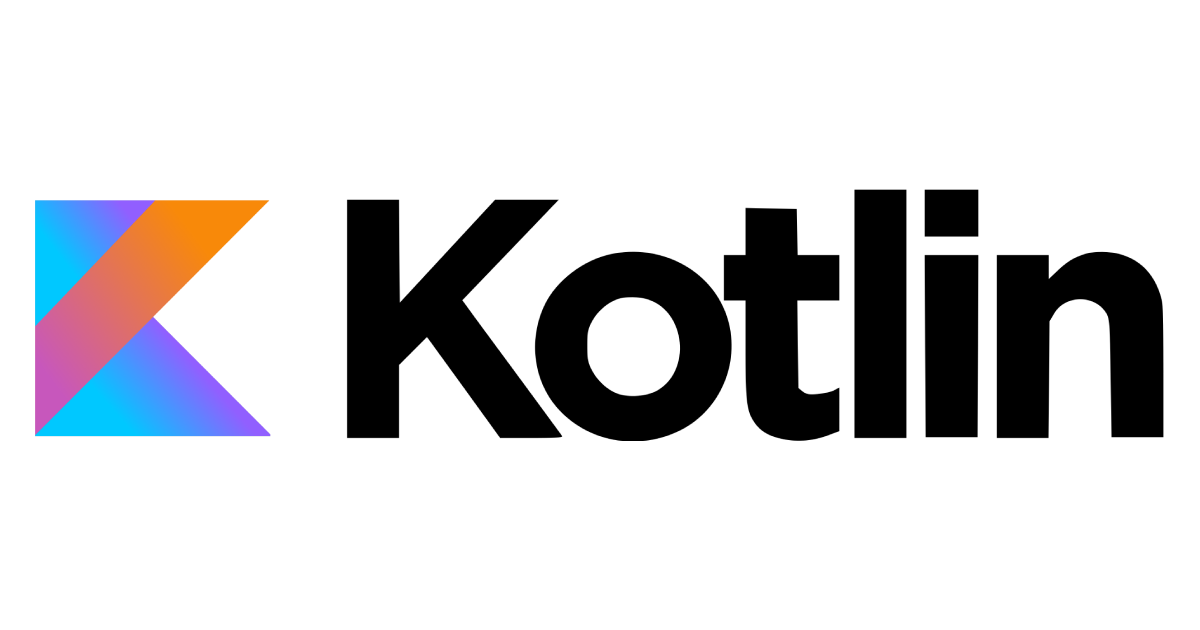
Kotlin is a modern, statically typed programming language that has gained popularity for its concise syntax and safety features. In this blog post, we will walk through a simple Kotlin program to understand its basic concepts and syntax.
The Kotlin Program
class Hello {
companion object {
@JvmStatic
fun main(args: Array) {
// Method call and print result
println("The sum is ${add(5, 10, 5)}")
The entry point of a Kotlin program is the main
function. In this program, it is defined inside a companion object with the @JvmStatic
annotation, which makes it a static method. The println
function is used to print the result of the add
method.
// Variables in Kotlin
// Always starts with capital letter unless using Java for both primitive and non-primitive types
// Int, String, Boolean, Float, Double, Long, Char, Byte, Short
var a: Int = 13
var b: String = "Hello"
var c: Boolean = true
var d: Float = 13.0f
var e: Double = 13.0
var f: Long = 13
var g: Char = 'A'
var h: Byte = 13
var i: Short = 13
// println(a)
Kotlin supports both mutable and immutable variables. Mutable variables are declared with var
and can be reassigned, while immutable variables are declared with val
and cannot be reassigned after their initial assignment. Here are examples of various types of variables declared in Kotlin:
// Difference Between Var & Val
// Val is used as final keyword like in Java & Var value can be reassigned
// Val can't be overridden & Var can be overridden
val j = 13
// j=135 // Uncommenting this line will cause an error because val cannot be reassigned
// println(j)
In Kotlin, var
is used to declare mutable variables, which means their value can be changed later. val
, on the other hand, is used to declare read-only variables (similar to final
in Java), and their value cannot be changed once assigned.
// Conditional Statements in Kotlin
// If, Else, When, For, While, Do While, Break, Continue, Try, Catch, Finally, Throw, Try
// If
var num1 = 100
var num2 = 200
// Using if-else expression
var msg = if (num1 > num2) "num1 is greater than num2" else "num1 is less than or equal to num2"
println(msg)
// An example of an if-else ladder (currently incomplete in the original code)
if (num1 > 100) {
println("num1 is greater than 100")
} else if (num1 == 100) {
println("num1 is equal to 100")
} else {
println("num1 is less than 100")
}
Kotlin supports traditional conditional statements like if
and else
. Here's an example of an if-else
expression:
// When
when(num1){
1 -> println("One")
2 -> println("Two")
3 -> println("Three")
100 -> println("100 h yeh")
else -> println("Invalid number")
}
The when
expression in Kotlin can be used as a replacement for the switch statement in Java. It allows for a more readable and concise syntax when dealing with multiple conditions.
//For and For Each
//Until is use to print 1 less value and downTo is use to decrement the value
var num3=0
for(i in 1..10 step 2){
println("no is ${num3++}")
}
Kotlin provides various types of loops to iterate over ranges, arrays, or other collections. Here's an example of a for
loop that iterates from 1 to 10 with a step of 2, printing the value of num3
on each iteration.
//While
var num4=10
while(num4<20 code="" is="" no="" num4="" println="">20>
This while
loop continues to execute as long as num4
is less than 20, incrementing and printing num4
on each iteration.
//Do While
var num5=10
do{
println("no is ${num5++}")
}while(num5<20 code="">20>
This do-while
loop executes at least once and continues to execute as long as num5
is less than 20, incrementing and printing num5
on each iteration.
// Methods in Kotlin
// Method for addition of two numbers
fun add(a: Int, b: Int): Int {
println("Addition of two numbers")
return a + b
}
// Method overloading for addition of three numbers
fun add(a: Int, b: Int, c: Int): Int {
println("Addition of three numbers")
return a + b + c
}
Kotlin supports defining functions, including overloaded functions. Here, we have two versions of the add
function: one that takes two parameters and another that takes three. The first method adds two integers, while the second method adds three integers. Both methods print a message before returning the result.
Conclusion
This simple Kotlin program demonstrates the basic syntax and features of the language, including variable declarations, conditional statements, loops, and methods. Kotlin's concise and expressive syntax makes it a powerful language for modern programming, especially for Android development.